Description
jade4j's intention is to be able to process jade templates in Java without the need of a JavaScript environment, while being fully compatible with the original jade syntax.
JADE alternatives and similar libraries
Based on the "Utility" category.
Alternatively, view JADE alternatives based on common mentions on social networks and blogs.
-
Dex
Dex : The Data Explorer -- A data visualization tool written in Java/Groovy/JavaFX capable of powerful ETL and publishing web visualizations. -
Stfalcon Fixturer
Utility for developers and QAs what helps minimize time wasting on writing the same data for testing over and over again. Made by Stfalcon -
Apache Commons
Provides different general purpose functions like configuration, validation, collections, file upload or XML processing.
WorkOS - The modern identity platform for B2B SaaS
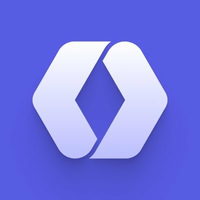
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of JADE or a related project?
Popular Comparisons
README
Attention: jade4j is now pug4j
In alignment with the javascript template engine we renamed jade4j to pug4j. You will find it under https://github.com/neuland/pug4j This is also a new release which supports almost the entire pug 2 syntax.
Please report pug4j issues in the new repository.
jade4j - a jade implementation written in Java
jade4j's intention is to be able to process jade templates in Java without the need of a JavaScript environment, while being fully compatible with the original jade syntax.
Contents
- Example
- Syntax
- Usage
- Simple static API
- Full API
- Expressions
- Reserved Words
- Framework Integrations
- Breaking Changes
- Authors
- License
Example
index.jade
doctype html
html
head
title= pageName
body
ol#books
for book in books
if book.available
li #{book.name} for #{book.price} €
Java model
List<Book> books = new ArrayList<Book>();
books.add(new Book("The Hitchhiker's Guide to the Galaxy", 5.70, true));
books.add(new Book("Life, the Universe and Everything", 5.60, false));
books.add(new Book("The Restaurant at the End of the Universe", 5.40, true));
Map<String, Object> model = new HashMap<String, Object>();
model.put("books", books);
model.put("pageName", "My Bookshelf");
Running the above code through String html = Jade4J.render("./index.jade", model)
will result in the following output:
<!DOCTYPE html>
<html>
<head>
<title>My Bookshelf</title>
</head>
<body>
<ol id="books">
<li>The Hitchhiker's Guide to the Galaxy for 5,70 €</li>
<li>The Restaurant at the End of the Universe for 5,40 €</li>
</ol>
</body>
</html>
Syntax
We have put up an interactive jade documentation.
See also the original visionmedia/jade documentation.
Usage
via Maven
As of release 0.4.1, we have changed maven hosting to sonatype. Using Github Maven Repository is no longer required.
Please be aware that we had to change the group id from 'de.neuland' to 'de.neuland-bfi' in order to meet sonatype conventions for group naming.
Just add following dependency definitions to your pom.xml
.
<dependency>
<groupId>de.neuland-bfi</groupId>
<artifactId>jade4j</artifactId>
<version>1.3.2</version>
</dependency>
Build it yourself
Clone this repository ...
git clone https://github.com/neuland/jade4j.git
... build it using maven
...
cd jade4j
mvn install
... and use the jade4j-1.x.x.jar
located in your target directory.
Simple static API
Parsing template and generating template in one step.
String html = Jade4J.render("./index.jade", model);
If you use this in production you would probably do the template parsing only once per template and call the render method with different models.
JadeTemplate template = Jade4J.getTemplate("./index.jade");
String html = Jade4J.render(template, model);
Streaming output using a java.io.Writer
Jade4J.render(template, model, writer);
Full API
If you need more control you can instantiate a JadeConfiguration
object.
JadeConfiguration config = new JadeConfiguration();
JadeTemplate template = config.getTemplate("index");
Map<String, Object> model = new HashMap<String, Object>();
model.put("company", "neuland");
config.renderTemplate(template, model);
Caching
The JadeConfiguration
handles template caching for you. If you request the same unmodified template twice you'll get the same instance and avoid unnecessary parsing.
JadeTemplate t1 = config.getTemplate("index.jade");
JadeTemplate t2 = config.getTemplate("index.jade");
t1.equals(t2) // true
You can clear the template and expression cache by calling the following:
config.clearCache();
For development mode, you can also disable caching completely:
config.setCaching(false);
Output Formatting
By default, Jade4J produces compressed HTML without unneeded whitespace. You can change this behaviour by enabling PrettyPrint:
config.setPrettyPrint(true);
Jade detects if it has to generate (X)HTML or XML code by your specified doctype.
If you are rendering partial templates that don't include a doctype jade4j generates HTML code. You can also set the mode
manually:
config.setMode(Jade4J.Mode.HTML); // <input checked>
config.setMode(Jade4J.Mode.XHTML); // <input checked="true" />
config.setMode(Jade4J.Mode.XML); // <input checked="true"></input>
Filters
Filters allow embedding content like markdown
or coffeescript
into your jade template:
script
:coffeescript
sayHello -> alert "hello world"
will generate
<script>
sayHello(function() {
return alert("hello world");
});
</script>
jade4j comes with a plain
and cdata
filter. plain
takes your input to pass it directly through, cdata
wraps your content in <![CDATA[...]]>
. You can add your custom filters to your configuration.
config.setFilter("coffeescript", new CoffeeScriptFilter());
To implement your own filter, you have to implement the Filter
Interface. If your filter doesn't use any data from the model you can inherit from the abstract CachingFilter
and also get caching for free. See the neuland/jade4j-coffeescript-filter project as an example.
Helpers
If you need to call custom java functions the easiest way is to create helper classes and put an instance into the model.
public class MathHelper {
public long round(double number) {
return Math.round(number);
}
}
model.put("math", new MathHelper());
Note: Helpers don't have their own namespace, so you have to be careful not to overwrite them with other variables.
p= math.round(1.44)
Model Defaults
If you are using multiple templates you might have the need for a set of default objects that are available in all templates.
Map<String, Object> defaults = new HashMap<String, Object>();
defaults.put("city", "Bremen");
defaults.put("country", "Germany");
defaults.put("url", new MyUrlHelper());
config.setSharedVariables(defaults);
Template Loader
By default, jade4j searches for template files in your work directory. By specifying your own FileTemplateLoader
, you can alter that behavior. You can also implement the TemplateLoader
interface to create your own.
TemplateLoader loader = new FileTemplateLoader("/templates/", "UTF-8");
config.setTemplateLoader(loader);
Expressions
The original jade implementation uses JavaScript for expression handling in if
, unless
, for
, case
commands, like this
- var book = {"price": 4.99, "title": "The Book"}
if book.price < 5.50 && !book.soldOut
p.sale special offer: #{book.title}
each author in ["artur", "stefan", "michael"]
h2= author
As of version 0.3.0, jade4j uses JEXL instead of OGNL for parsing and executing these expressions.
We decided to switch to JEXL because its syntax and behavior is more similar to ECMAScript/JavaScript and so closer to the original jade.js implementation. JEXL runs also much faster than OGNL. In our benchmark, it showed a performance increase by factor 3 to 4.
We are using a slightly modified JEXL version which to have better control of the exception handling. JEXL now runs in a semi-strict mode, where non existing values and properties silently evaluate to null
/false
where as invalid method calls lead to a JadeCompilerException
.
Reserved Words
JEXL comes with the three builtin functions new
, size
and empty
. For properties with this name the .
notation does not work, but you can access them with []
.
- var book = {size: 540}
book.size // does not work
book["size"] // works
You can read more about this in the JEXL documentation.
Framework Integrations
- neuland/spring-jade4j jade4j for Spring.
- jooby-jade jade4j for Jooby.
- vertx-web jade4j for Vert.X
Breaking Changes
1.3.1
- Fixed a mayor scoping bug in loops. Use this version and not 1.3.0
1.3.0
- setBasePath has been removed from JadeConfiguration. Set folderPath on FileTemplateLoader instead.
- Scoping of variables in loops changed, so its more in line with jade. This could break your template.
1.2.0
- Breaking change in filter interface: if you use filters outside of the project, they need to be adapted to new interface
1.0.0
In Version 1.0.0 we added a lot of features of JadeJs 1.11. There are also some Breaking Changes:
- Instead of 'id = 5' you must use '- var id = 5'
- Instead of 'h1(attributes, class = "test")' you must use 'h1(class= "test")&attributes(attributes)'
- Instead of '!!! 5' you must use 'doctype html'
- Jade Syntax for Conditional Comments is not supported anymore
- Thanks to rzara for contributing to issue-108
Authors
- Artur Tomas / atomiccoder
- Stefan Kuper / planetk
- Michael Geers / naltatis
- Christoph Blömer / chbloemer
Special thanks to TJ Holowaychuk the creator of jade!
License
The MIT License
Copyright (C) 2011-2019 neuland Büro für Informatik, Bremen, Germany
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*Note that all licence references and agreements mentioned in the JADE README section above
are relevant to that project's source code only.