Description
VerbalExpressions is a Java library that helps to construct difficult regular expressions.
JavaVerbalExpressions alternatives and similar libraries
Based on the "Utility" category.
Alternatively, view JavaVerbalExpressions alternatives based on common mentions on social networks and blogs.
-
Dex
Dex : The Data Explorer -- A data visualization tool written in Java/Groovy/JavaFX capable of powerful ETL and publishing web visualizations. -
Stfalcon Fixturer
Utility for developers and QAs what helps minimize time wasting on writing the same data for testing over and over again. Made by Stfalcon -
Apache Commons
Provides different general purpose functions like configuration, validation, collections, file upload or XML processing.
InfluxDB - Power Real-Time Data Analytics at Scale
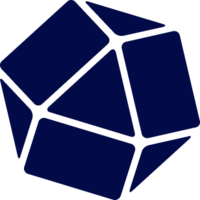
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of JavaVerbalExpressions or a related project?
README
JavaVerbalExpressions
VerbalExpressions is a Java library that helps to construct difficult regular expressions.
Getting Started
Maven Dependency:
<dependency>
<groupId>ru.lanwen.verbalregex</groupId>
<artifactId>java-verbal-expressions</artifactId>
<version>1.5</version>
</dependency>
You can use SNAPSHOT dependency with adding to pom.xml
:
<repositories>
<repository>
<id>ossrh</id>
<url>https://oss.sonatype.org/content/repositories/snapshots</url>
</repository>
</repositories>
Examples
VerbalExpression testRegex = VerbalExpression.regex()
.startOfLine().then("http").maybe("s")
.then("://")
.maybe("www.").anythingBut(" ")
.endOfLine()
.build();
// Create an example URL
String url = "https://www.google.com";
// Use VerbalExpression's testExact() method to test if the entire string matches the regex
testRegex.testExact(url); //True
testRegex.toString(); // Outputs the regex used:
// ^(?:http)(?:s)?(?:\:\/\/)(?:www\.)?(?:[^\ ]*)$
VerbalExpression testRegex = VerbalExpression.regex()
.startOfLine().then("abc").or("def")
.build();
String testString = "defzzz";
//Use VerbalExpression's test() method to test if parts if the string match the regex
testRegex.test(testString); // true
testRegex.testExact(testString); // false
testRegex.getText(testString); // returns: def
Builder can be cloned:
VerbalExpression regex = regex(regex().anything().addModifier('i')).endOfLine().build();
Or can be used in another regex:
VerbalExpression.Builder digits = regex().capt().digit().oneOrMore().endCapt().tab();
VerbalExpression regex2 = regex().add(digits).add(digits).build();
Feel free to use any predefined char groups:
regex().wordChar().nonWordChar()
.space().nonSpace()
.digit().nonDigit()
Define captures:
String text = "aaabcd";
VerbalExpression regex = regex()
.find("a")
.capture().find("b").anything().endCapture().then("cd").build();
regex.getText(text) // returns "abcd"
regex.getText(text, 1) // returns "b"
More complex examples
Other implementations
You can view all implementations on VerbalExpressions.github.io
[ Javascript - PHP - Python - C# - Objective-C - Ruby - Groovy - Haskell - C++ - ... (moarr) ]
Project released with travis
With help of this tutorial: https://dracoblue.net/dev/uploading-snapshots-and-releases-to-maven-central-with-travis/